A Briefcase Full of Code - War is a Failure of Diplomacy
Sunday, 03/13/2005
Definitely LISPy
Topic: Daily Info
Here's that wicked homework I was working on.
Note: I absolve myself of any guarantees about this code, except that is you use it in a homework assignment without giving me full credit, you are plagiarizing my code. I am coverning my ass against academic dishonesty, as this assingment was due Friday, March 11, 2005.Now that those pleasantries are over, on to some LISPy goodness:
;;Builds a structure from which we can glena information about the minimum
;;spanning tree
(defun build-mst-struct (map)
(if (eql map '())
'()
(cons (cons (caar map) (cons 'INFINITY 'NOPRED))
(build-mst-struct (cdr map)))))
;;Determines if some symbol is in the map, used in the actual Prim's MST
;;algorithm
(defun in-map-list (map symbol)
(if (eql map '())
'()
(if (eql (caar map) symbol)
T
(in-map-list (cdr map) symbol))))
;;Sets both the key and the predecessor of a node in one shot to save excess
;;tree traversal
(defun set-key-pred-helper (node keyval predval)
(setf (cadr node) keyval)
(setf (cddr node) predval)
keyval)
;;Finds symbol in mst-struct and sets its key=keyval and predecssor=predval
(defun set-key-pred (symbol keyval predval mst-struct)
(if (eql mst-struct '())
'()
(if (eql (caar mst-struct) symbol)
(set-key-pred-helper (car mst-struct) keyval predval)
(set-key-pred symbol keyval predval (cdr mst-struct)))))
;;Used to extract the adjaceny list from the topmost node of the map
(defun pull-adj-list (map)
(car (cddr (car map))))
;;Determines the weight of the edge of the first node in the adjacency list
(defun adj-weight (adj-list)
(cdar adj-list))
;;Retrieves the key associated with symbol in the mst-struct
(defun get-key (symbol mst-struct)
(if (eql mst-struct '())
'()
(if (eql (caar mst-struct) symbol)
(cadar mst-struct)
(get-key symbol (cdr mst-struct)))))
;;My work-around since I didn't know if LISP had the concept of infinity
;;I have defined my own infinity symbol and this checks first to see if
;;keyval is infinite (from Prim's setup) and if not, compares against the
;;passed in weight
(defun weight-less-than-key (weight keyval)
(if (eql keyval 'INFINITY)
T
(< weight keyval)))
(defun set-and-loop (symbol keyval predval map mst-struct adj-list)
(set-key-pred symbol
keyval
predval
mst-struct)
(proc-adj-list mst-struct map (cdr adj-list)))
;;This is the inner loop of Prim's algorithm
(defun proc-adj-list (mst-struct map adj-list)
(if (eql adj-list '())
'()
;;Checking if the next node in the adjanceny list is still in Q
;;If not, we can move onto the next node
(if (not (in-map-list map (caar adj-list)))
(proc-adj-list mst-struct map (cdr adj-list))
;;The node in the adjancency list was in the map still, so no we
;;Do the meat of Prim's, check w(u,v) < key[v]
(if (weight-less-than-key (adj-weight adj-list)
(get-key (caar adj-list) mst-struct))
(set-and-loop (caar adj-list)
(adj-weight adj-list)
(caar map)
map
mst-struct
adj-list)))))
;;This is the main worker of Prim's Algorithm
(defun mst-worker-loop (mst-struct map)
(proc-adj-list mst-struct map (pull-adj-list map))
(mst-worker mst-struct (cdr map)))
(defun mst-worker (mst-struct map)
(if (eql map '())
mst-struct
(mst-worker-loop mst-struct map)))
;;Finally the main mst
(defun mst (map)
(setf mst-struct (build-mst-struct map))
(mst-worker mst-struct map))
(defun mst-cost (to-visit map)
(defun mst-helper (to-visit mst-struct)
(if (eql to-visit '())
0
(+ (get-key (car to-visit) mst-struct)
(mst-helper (cdr to-visit) mst-struct))))
(mst-helper to-visit (mst map)))
Crazy, ain't it?
Friday, 03/11/2005
Stuff and junk and stuff and junk
Topic: Daily Info
Hmmm, access to a
Cray X1. Not as cool as
Blue Gene or
The Columbia Installation, but seriously cool anyways, and about the closest I'm going to come to a "real" (they make our little
Unisys Box look like an Atari 800) supercomputer until the lab starts working with
PittsburghNot that I'm really into writing that kind of code anymore. My interest in large parallel computers is quickly waning. I find AI and networking to be more fun. Anyone can work in AI and networking; only goverments, univeristies and large corporations can do large parallel computing.
Yup, about 6 hours of actual code and paper writing combined with about 15 or 16 hours of straight-up thought on how to take some well known algorithms and do them effeciently in LISP. So all told, 24 hours of work on one assigment. And call me weird, but I actually enjoyed doing it. It was fun, like programming back in freshman year was fun. But instead of very simple programs, I'm now writing nice and complex programs that do something interesting. I did kind of finish up in a half-assed manner, and now the program has a slightly larger runtime (about 0.01 seconds longer on average). If I was willing to go back in tomorrow, I think I may have a fix that would drop the run-time penalty. But otherwise it did work: it made the program much more memory effecient and it takes fewer trials to find the goal. Worked the first time after clearing up some small glitches (you write 600-700 lines of LISP and not make one or two errors).
So yeah, I think AI and something with networks will be my major-emphasis. It's just too much fun.
And I wish I could sleep, I really do. I finally got to bed around 4 yesterday and then got up at 6, and did my marathon. And add on the extended discussion about systems software at work (I'll go into it later) and I'm just about fried.
The plan for tomorrow is to clean up a few slushy stains in the car and shop about for some new boots.
Later-o.
Thursday, 03/10/2005
Nifty Shit, Man!
Topic: Daily Info
Sweet! I just found this
little deally that will let me hook up my Mp3 player to my car's stereo without that goofy Player-to-Tape arrangement.
Best part about it? No changing CD's. With my 5 GB (which leaves around 15 GB of free space on the player) of music, I currently have over 3 days of playtime, and that's not even half my collection of CD's (fair use RIAA, I paid you for the damn CD's already, you got your money, back off).
So I think I'll order one of these babies, some nice shielded cables to bring the connectors up near the dash (probably under the cup-holder/ash-tray thing) and out this on my list of spring break projects.
Oh yeah.
Monday, 02/28/2005
Shoes, a bit more TV junk, FAFSA, et cetera
Topic: Daily Info
Blessed are the stained glass, window pane glass.
Blessed is the church service makes me nervous
Blessed are the penny rookers, Cheap hookers, Groovy lookers.
O Lord, Why have you forsaken me?
I have tended my own garden
Much too long.Blessed, Simon and Garfunkel
So the "pump" shoes are back again. I had a pair once, long, long ago, and they sucked. They lasted a year before I had shredded them to pieces.
Now the Vans I graduated in from high school, those were some shoes. Two years I beat the living hell out of those shoes, many, many miles I walked. I'd probably still be wearing them if I hadn't decided to hang them up after graduation.
Anyways. I'd go further into my no TV pledge with a bigger elaboration and explanation, but I don't rightly feel like it. If I have time this spring break or this summer, I'll go over it in greater detail and apply some heavy logic to it. Someone remind me.
Only one more FAFSA dealy to fill out. Which is nice, since I find the thing to be a massive pain in the ass. I know it's a government website, but for how many people use it, they could drastically simplify the process.
On a side note, I have made some good progress on the paradigm essay. Pushing about 15 pages at the moment, yet I haven't even covered half of what I want to cover with it.
Monday, 02/21/2005
Thbbbbbppppptttttt
Topic: Daily Info
Ebeh ...
I want to get back to writing my two books (the one about computers and the sci-fi book), but I never seem to have any damn time to do it in. By the time I get caught up with homework, I'm dead tired or working or something generally menial and uninteresting.
Speaking of the computer book, I think I'll post one of the appendices as an independent article here first so I can flesh it out further. In short, it's about the abuse and misuse of paradigms in the world of computers, a bit of my feelings on languages, their misuse, and agnosticism of language (although I may spin that one into it's own appendix essay).
On other fronts, I got the server back up and running which makes me fell better. It is my main backup device (holds the CVS server as well as a huge data-sink). It is still named Jet. Now if I could find time to get Bebop up and installed and Ophelia (the name I chose for the other one) to at least farging boot (although if it remains dead, it'd be awfully close to its namesake).
Yeah, that's it. You can carry on with your business now.
Thursday, 02/17/2005
Know
Topic: Daily Info
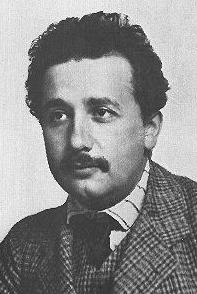
One hundred years ago, this man changed the face of science. Not the strange old Princeton sage that people market the hell out of, this young man. He was just five years older than I am now. And yet, no one person has topped his accomplishments in that six-month span.
Four simple papers, and everything changed. Yes, even then he was still a pacifist, great humorist, and a humanitarian. But he was a man struggling to survive, wokring long days and scribbling into the night, pulling hints from his cup of tea (stirring a cup of tea gave him inspiration to give a proof of Brownian motion, which was just warming up for Albert).
So don't quote Einstein like its some mystic anti-science thing. For Einstein, using science to understand the universe was looking directly at god. Einstein just wanted to have an idea of what god was thinking. "God does not play dice" was Einstein's conviction not in a divine plan, but rather that god played by nice, regular rules.
Anyway, remember that young man at 26, not the old sage at 72 when you think of quiet genius and studious thought. Think of the old sage when you want to think of humility and humanity. For more Einstein, try here.
Oh yeah. Guess which city is the most tech-savvy in the country? No, not in California. It's Minneapolis. Why? Go grab a copy of PopSci and find out why. Snd it really doesn't suprise me as much as it surprised Paul and David.
Saturday, 02/12/2005
Numbing
Topic: Daily Info
My mind is set on overdrive
The clock is laughing in my face
A crooked spine
My senses dulled
Passed the point of delirium
On my own... here we go Brain Stew, Green Day
Yeah, I'll try to never do that again. That was fucking intense. It's one thing to stay up all night and then fuck about at home, but another to go to classes, take notes and participate in discussions. Killer. And to top it off, I think my essay was a total piece of shit.
I was getting pretty bad by the end of the night, as anyone who talked to me could attest to. By the time I went to bed, I was starting to lose chunks of time as large as ten minutes, just randomly interspersed. I vaguely remember having leftover beef stroganov for dinner, then some show, Good Eats, then about ten minutes of Family Guy, then getting undressed and waking up on Friday at 12:00. I still feel off today, a little discombobulated, like I'm a whole day behind everyone else. I dunno.
Got three assignments due by Tuesday, but if I grind at them today, Sunday and Monday, I should be fine. The one is basically done and I'm doing it in a group, and as much as I hate shifting a bit of work to them, I'll more than make up for it later. Besides, I should get some kind of break for taking 12 credits worth of graduate level classes.
Oh yeah, if you have a fairly new computer (GHz clock or better), a bunch of RAM, and leave your computer on for long stretches of time, look into these following links. Maybe we can help find a cure for some disease.
Folding@HomeUnited DevicesAnyway, I might have something more to write tomorrow, but in all likelihood, not really.
And a quick apology to all those people that I was an ass to on Thursday and Friday.
EOF
Thursday, 02/10/2005
Forced Insomnia
Topic: Daily Info
Yup, I have now been up since around 8:45 am on Wednesday. Going on 20 hours and 04 minutes by my clock. My fault for putting off this stupid paper until today. I mostly finished it around three, three-thirty, but considering I get up at six, I figured I may as well stay up the whole time and just go home early tomorrow. Better to stay up, feel less like shit. Ah well, at least I can catch up on sleep for Friday and then try to stay on top of things more for next time.
Yeesh, my mind is already wandering about. It's gonna be tough slogging through until I hand in this paper.
Wish me luck.
Tuesday, 02/08/2005
New Year
Topic: Daily Info
Anbon reminded me just a bit ago, Happy New Year to my Chinese friends out there.
Saturday, 01/29/2005
A short little story
Topic: Daily Info
We're the regulators that de-regulate
We're the animators that de-animate
We're the propogators of all genocide
Burning through the world's resources that we cannot hide
Fool in denial
We're the cruel regulators smoking
Cigaro, cigaro, cigaro
Fool in denial
We're the cruel regulators smoking
Cigaro, cigaro, cigaroCigaro, System of a Down
Another thing,
here's a short story I started on Thursday and finished just a little while ago. It's only about 7 pages of text (discounting the title page and copyright page), and is around 80 KB in size so it shouldn't break your bandwidth to download. The layout is setup to be printed and bound into a book, which explains why the pages look slightly off-kilter, this is on purpose.
Read and enjoy.
Newer | Latest | Older